处理流之一——缓冲流-字节型
1 2 3 4 5 6 7 8 9 10 11 12
| 处理流之一:缓冲流的使用 * * 1.缓冲流: * BufferedInputStream * BufferedOutputStream * BufferedReader * BufferedWriter * * 2.作用:提供流的读取、写入的速度 * 提高读写速度的原因:内部提供了一个缓冲区 * * 3. 处理流,就是“套接”在已有的流的基础上。
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187
| public class BufferedTest {
@Test public void BufferedStreamTest() throws FileNotFoundException { BufferedInputStream bis = null; BufferedOutputStream bos = null;
try { File srcFile = new File("爱情与友情.jpg"); File destFile = new File("爱情与友情3.jpg"); FileInputStream fis = new FileInputStream((srcFile)); FileOutputStream fos = new FileOutputStream(destFile); bis = new BufferedInputStream(fis); bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[10]; int len; while((len = bis.read(buffer)) != -1){ bos.write(buffer,0,len);
} } catch (IOException e) { e.printStackTrace(); } finally { if(bos != null){ try { bos.close(); } catch (IOException e) { e.printStackTrace(); }
} if(bis != null){ try { bis.close(); } catch (IOException e) { e.printStackTrace(); }
}
}
}
public void copyFileWithBuffered(String srcPath,String destPath){ BufferedInputStream bis = null; BufferedOutputStream bos = null;
try { File srcFile = new File(srcPath); File destFile = new File(destPath); FileInputStream fis = new FileInputStream((srcFile)); FileOutputStream fos = new FileOutputStream(destFile); bis = new BufferedInputStream(fis); bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[1024]; int len; while((len = bis.read(buffer)) != -1){ bos.write(buffer,0,len); } } catch (IOException e) { e.printStackTrace(); } finally { if(bos != null){ try { bos.close(); } catch (IOException e) { e.printStackTrace(); }
} if(bis != null){ try { bis.close(); } catch (IOException e) { e.printStackTrace(); }
}
} }
@Test public void testCopyFileWithBuffered(){ long start = System.currentTimeMillis();
String srcPath = "C:\\Users\\Administrator\\Desktop\\01-视频.avi"; String destPath = "C:\\Users\\Administrator\\Desktop\\03-视频.avi";
copyFileWithBuffered(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start)); }
@Test public void testBufferedReaderBufferedWriter(){ BufferedReader br = null; BufferedWriter bw = null; try { br = new BufferedReader(new FileReader(new File("dbcp.txt"))); bw = new BufferedWriter(new FileWriter(new File("dbcp1.txt")));
String data; while((data = br.readLine()) != null){
bw.write(data); bw.newLine();
}
} catch (IOException e) { e.printStackTrace(); } finally { if(bw != null){
try { bw.close(); } catch (IOException e) { e.printStackTrace(); } } if(br != null){ try { br.close(); } catch (IOException e) { e.printStackTrace(); }
} }
}
}
|
练习一

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49
| @Test public void test1() {
FileInputStream fis = null; FileOutputStream fos = null; try { fis = new FileInputStream("爱情与友情.jpg"); fos = new FileOutputStream("爱情与友情secret.jpg");
byte[] buffer = new byte[20]; int len; while ((len = fis.read(buffer)) != -1) { for (int i = 0; i < len; i++) { buffer[i] = (byte) (buffer[i] ^ 5); }
fos.write(buffer, 0, len); } } catch (IOException e) { e.printStackTrace(); } finally { if (fos != null) { try { fos.close(); } catch (IOException e) { e.printStackTrace(); }
} if (fis != null) { try { fis.close(); } catch (IOException e) { e.printStackTrace(); }
} }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| @Test public void test2() {
FileInputStream fis = null; FileOutputStream fos = null; try { fis = new FileInputStream("爱情与友情secret.jpg"); fos = new FileOutputStream("爱情与友情4.jpg");
byte[] buffer = new byte[20]; int len; while ((len = fis.read(buffer)) != -1) { for (int i = 0; i < len; i++) { buffer[i] = (byte) (buffer[i] ^ 5); }
fos.write(buffer, 0, len); } } catch (IOException e) { e.printStackTrace(); } finally { if (fos != null) { try { fos.close(); } catch (IOException e) { e.printStackTrace(); }
} if (fis != null) { try { fis.close(); } catch (IOException e) { e.printStackTrace(); }
} }
}
|
提示:(异或运算符)
练习二

1 2 3 4 5 6 7 8 9 10 11
| 练习3:获取文本上字符出现的次数,把数据写入文件 * * 思路: * 1.遍历文本每一个字符 * 2.字符出现的次数存在Map中 * * Map<Character,Integer> map = new HashMap<Character,Integer>(); * map.put('a',18); * map.put('你',2); * * 3.把map中的数据写入文件
|
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77
| public class WordCount {
@Test public void testWordCount() { FileReader fr = null; BufferedWriter bw = null; try { Map<Character, Integer> map = new HashMap<Character, Integer>();
fr = new FileReader("dbcp.txt"); int c = 0; while ((c = fr.read()) != -1) { char ch = (char) c; if (map.get(ch) == null) { map.put(ch, 1); } else { map.put(ch, map.get(ch) + 1); } }
bw = new BufferedWriter(new FileWriter("wordcount.txt"));
Set<Map.Entry<Character, Integer>> entrySet = map.entrySet(); for (Map.Entry<Character, Integer> entry : entrySet) { switch (entry.getKey()) { case ' ': bw.write("空格=" + entry.getValue()); break; case '\t': bw.write("tab键=" + entry.getValue()); break; case '\r': bw.write("回车=" + entry.getValue()); break; case '\n': bw.write("换行=" + entry.getValue()); break; default: bw.write(entry.getKey() + "=" + entry.getValue()); break; } bw.newLine(); } } catch (IOException e) { e.printStackTrace(); } finally { if (fr != null) { try { fr.close(); } catch (IOException e) { e.printStackTrace(); }
} if (bw != null) { try { bw.close(); } catch (IOException e) { e.printStackTrace(); }
} }
} }
|
处理流之二——转换流
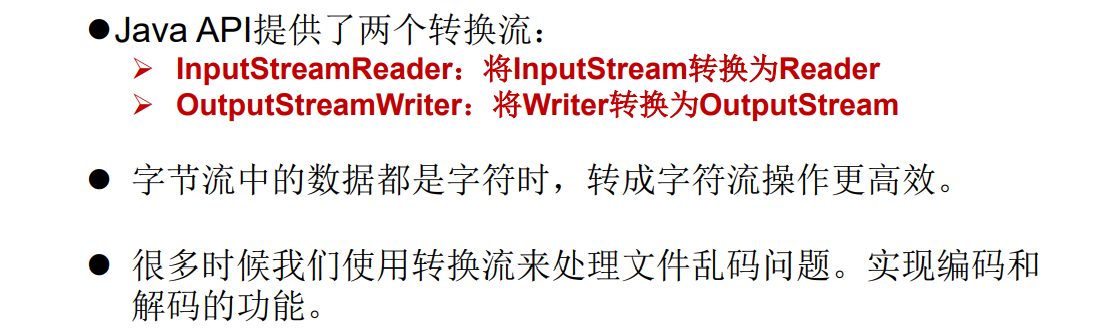
1 2 3 4 5 6 7 8
| 1.转换流:属于字符流 * InputStreamReader:将一个字节的输入流转换为字符的输入流 * OutputStreamWriter:将一个字符的输出流转换为字节的输出流 * * 2.作用:提供字节流与字符流之间的转换 * * 3. 解码:字节、字节数组 --->字符数组、字符串 * 编码:字符数组、字符串 ---> 字节、字节数组
|
补充
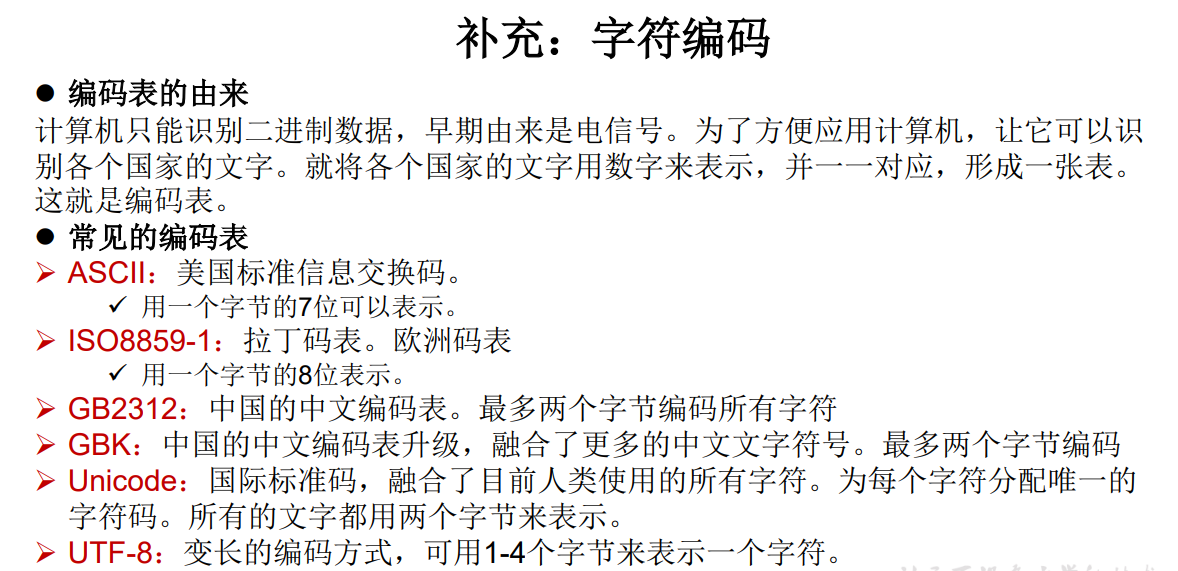
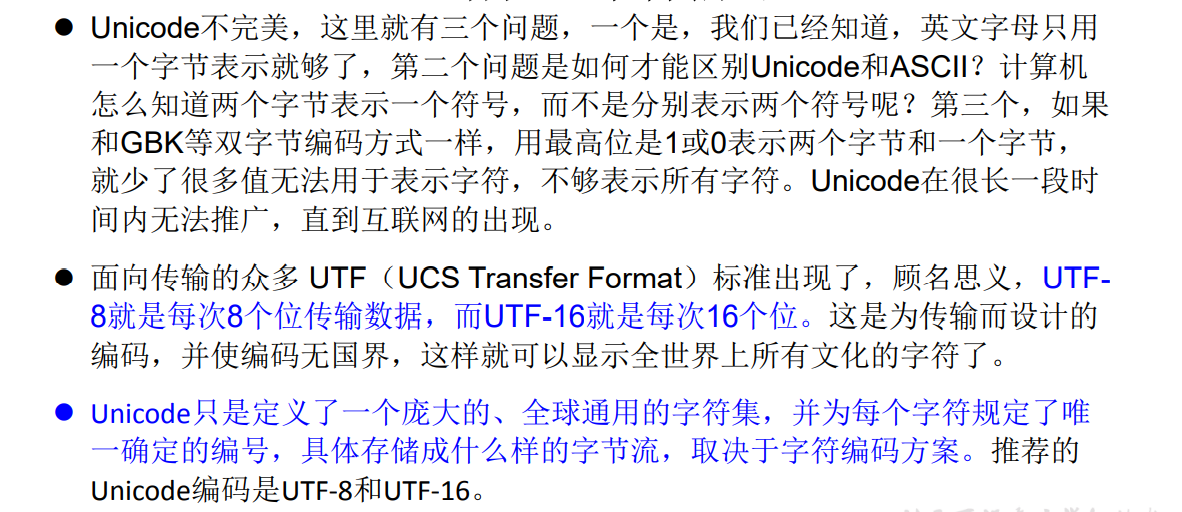
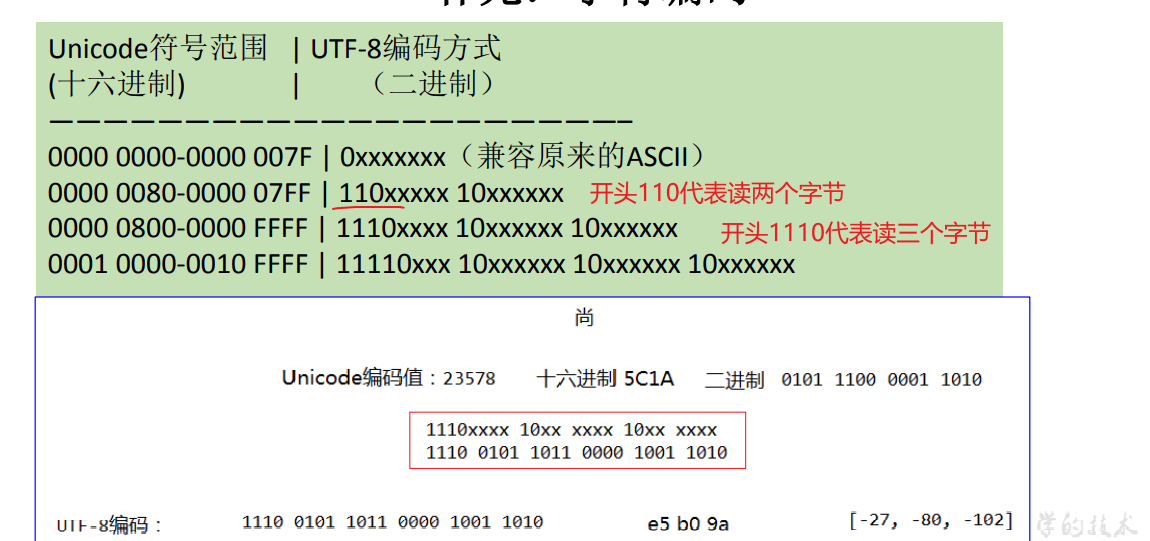
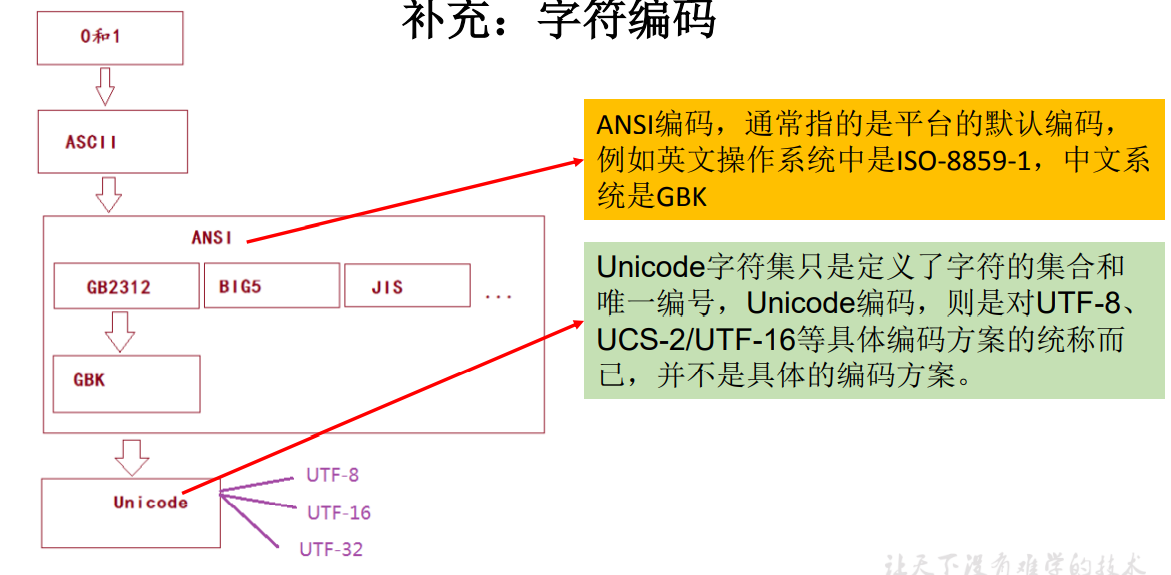
代码示例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
|
@Test public void test1() throws IOException {
FileInputStream fis = new FileInputStream("dbcp.txt");
InputStreamReader isr = new InputStreamReader(fis,"UTF-8");
char[] cbuf = new char[20]; int len; while((len = isr.read(cbuf)) != -1){ String str = new String(cbuf,0,len); System.out.print(str); }
isr.close();
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
@Test public void test2() throws Exception { File file1 = new File("dbcp.txt"); File file2 = new File("dbcp_gbk.txt");
FileInputStream fis = new FileInputStream(file1); FileOutputStream fos = new FileOutputStream(file2);
InputStreamReader isr = new InputStreamReader(fis,"utf-8"); OutputStreamWriter osw = new OutputStreamWriter(fos,"gbk");
char[] cbuf = new char[20]; int len; while((len = isr.read(cbuf)) != -1){ osw.write(cbuf,0,len); }
isr.close(); osw.close();
}
|