super关键字的使用
1.super理解为:父类的
2.super可以用来调用:属性、方法、构造器
3.super的使用:调用属性和方法
3.1 我们可以在子类的方法或构造器中。通过使用"super.属性"或"super.方法"的方式,显式的调用
父类中声明的属性或方法。但是,通常情况下,我们习惯省略"super."
3.2 特殊情况:当子类和父类中定义了同名的属性时,我们要想在子类中调用父类中声明的属性,则必须显式的
使用"super.属性"的方式,表明调用的是父类中声明的属性。
3.3 特殊情况:当子类重写了父类中的方法以后,我们想在子类的方法中调用父类中被重写的方法时,则必须显式的
使用"super.方法"的方式,表明调用的是父类中被重写的方法。
4.super调用构造器
4.1 我们可以在子类的构造器中显式的使用"super(形参列表)"的方式,调用父类中声明的指定的构造器
4.2 “super(形参列表)”的使用,必须声明在子类构造器的首行!
4.3 我们在类的构造器中,针对于”this(形参列表)”或”super(形参列表)”只能二选一,不能同时出现
4.4 在构造器的首行,没有显式的声明”this(形参列表)”或”super(形参列表)”,则默认调用的是父类中空参的构造器:super()
4.5 在类的多个构造器中,至少有一个类的构造器中使用了”super(形参列表)”,调用父类中的构造器
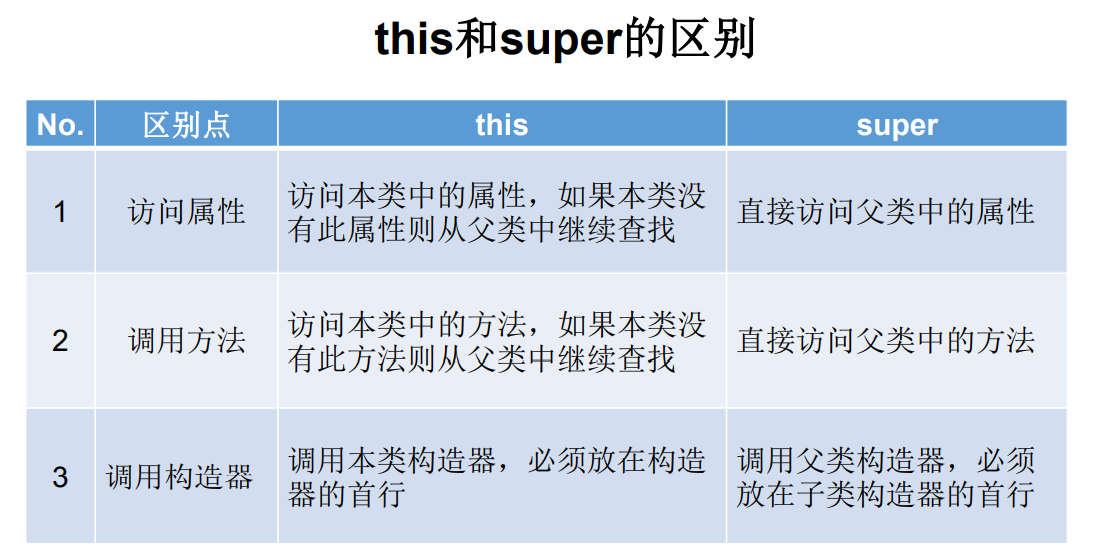
子类对象实例化的全过程
从结果上来看:(继承性)
子类继承父类以后,就获取了父类中声明的属性或方法。
创建子类的对象,在堆空间中,就会加载所有父类中声明的属性。
从过程上来看:
当我们通过子类的构造器创建子类对象时,我们一定会直接或间接的调用其父类的构造器,进而调用父类的父类的构造器,...
直到调用了java.lang.Object类中空参的构造器为止。正因为加载过所有的父类的结构,所以才可以看到内存中有
父类中的结构,子类对象才可以考虑进行调用。
明确:虽然创建子类对象时,调用了父类的构造器,但是自始至终就创建过一个对象,即为new的子类对象。
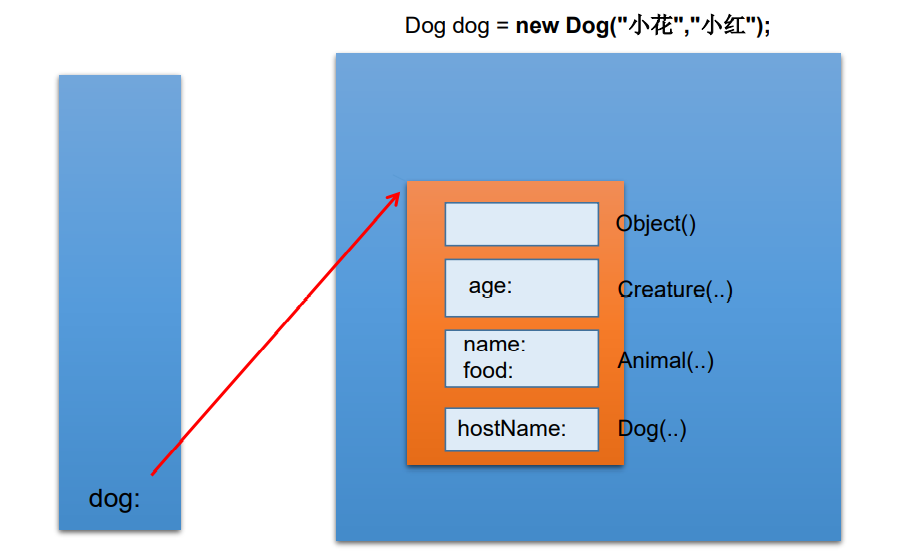
综合练习:
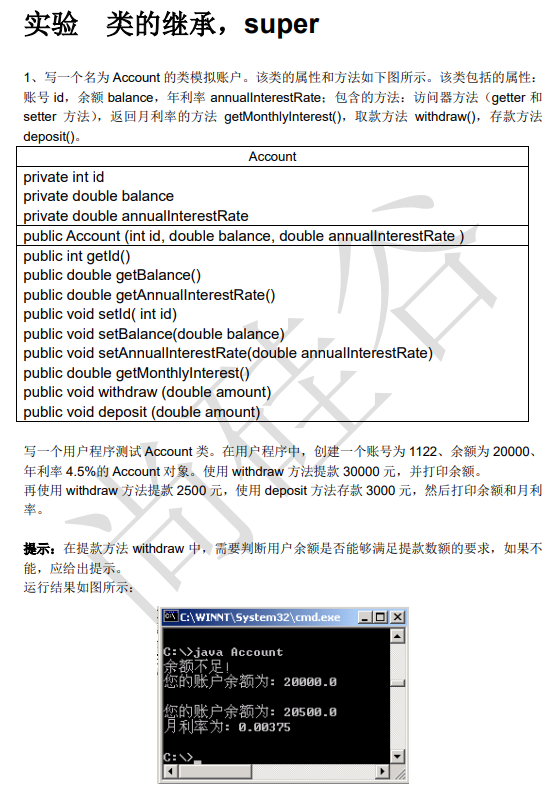
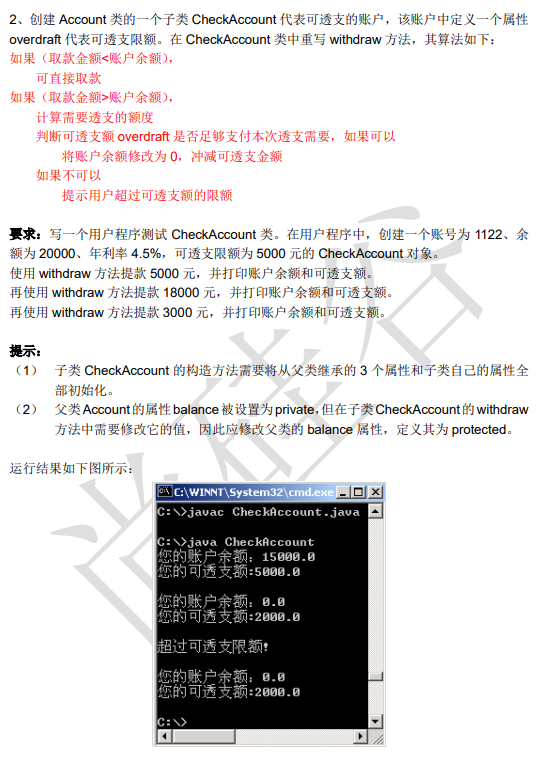
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| public class Account { private int id; private double balance; private double annualInterestRate; public Account(int id, double balance, double annualInterestRate) { super(); this.id = id; this.balance = balance; this.annualInterestRate = annualInterestRate; }
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public double getBalance() { return balance; }
public void setBalance(double balance) { this.balance = balance; }
public double getAnnualInterestRate() { return annualInterestRate; }
public void setAnnualInterestRate(double annualInterestRate) { this.annualInterestRate = annualInterestRate; } public double getMonthlyInterest(){ return annualInterestRate / 12; } public void withdraw (double amount){ if(balance >= amount){ balance -= amount; return; } System.out.println("余额不足"); } public void deposit (double amount){ if(amount > 0){ balance += amount; } }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
public class AccountTest { public static void main(String[] args) { Account acct = new Account(1122, 20000, 0.045); acct.withdraw(30000); System.out.println("您的账户余额为:" + acct.getBalance()); acct.withdraw(2500); System.out.println("您的账户余额为:" + acct.getBalance()); acct.deposit(3000); System.out.println("您的账户余额为:" + acct.getBalance()); System.out.println("月利率为:" + (acct.getMonthlyInterest() * 100) +"%"); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
|
public class CheckAccount extends Account{ private double overdraft; public CheckAccount(int id, double balance, double annualInterestRate,double overdraft){ super(id, balance, annualInterestRate); this.overdraft = overdraft; } public double getOverdraft() { return overdraft; }
public void setOverdraft(double overdraft) { this.overdraft = overdraft; }
@Override public void withdraw(double amount) { if(getBalance() >= amount){
super.withdraw(amount); }else if(overdraft >= amount - getBalance()){ overdraft -= (amount - getBalance());
super.withdraw(getBalance()); }else{ System.out.println("超过可透支限额!"); } }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
|
public class CheckAccountTest { public static void main(String[] args) { CheckAccount acct = new CheckAccount(1122, 20000, 0.045, 5000); acct.withdraw(5000); System.out.println("您的账户余额为:" + acct.getBalance()); System.out.println("您的可透支额度为:" + acct.getOverdraft()); acct.withdraw(18000); System.out.println("您的账户余额为:" + acct.getBalance()); System.out.println("您的可透支额度为:" + acct.getOverdraft()); acct.withdraw(3000); System.out.println("您的账户余额为:" + acct.getBalance()); System.out.println("您的可透支额度为:" + acct.getOverdraft()); } }
|